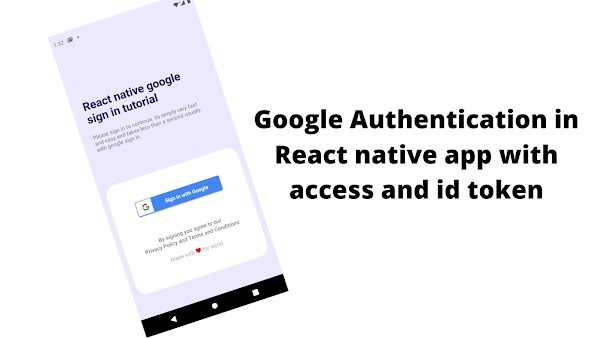
Building mobile applications with reactive native and Django is blazing fast at least for me. In this tutorial let us build a react native app with google authentication. If you are new to react native check out this guide to get started. This is a bare workflow, not an expo build since we need to tweak the android app source.
1. create a react native project
npx react-native init AwesomeProject
2. Install the Dependency package
yarn add @react-native-google-signin/google-signin
3. Create a new project in the firebase console.
(Do enter the android package name and SHA1 Debug certificate )
From your console settings download google-services.json file
Place this JSON file in Project/android/app/google-services.json
4. configuring android
open android/app/build.gradle and add this to the end of the file.
apply plugin: 'com.google.gms.google-services'
open android/build.gradle
add this to dependencies
classpath 'com.google.gms:google-services:4.3.10'
and set google play auth version in build script
buildscript{
ext {
buildToolsVersion = "30.0.2"
minSdkVersion = 21
compileSdkVersion = 30
targetSdkVersion = 30
ndkVersion = "21.4.7075529"
googlePlayServicesAuthVersion = "19.2.0" <--------------------------->
}
--------------------------->
5. Auth Screen
web client id is available in google services.json the one with client_type: 3
{ "client_id": "YOUR___ID__.apps.googleusercontent.com", "client_type": 3 }
Auth Screen Code
import React from 'react';
import {View, Text, StatusBar, StyleSheet, Image} from 'react-native';
import {SafeAreaView} from 'react-native-safe-area-context';
import {Button, Surface} from 'react-native-paper';
import MaterialCommunityIcons from 'react-native-vector-icons/MaterialCommunityIcons';
import Axios from 'axios';
import {inject, observer} from 'mobx-react';
import {
GoogleSignin,
GoogleSigninButton,
statusCodes,
} from '@react-native-google-signin/google-signin';
const Auth = props => {
const [loading, setLoading] = React.useState(false);
GoogleSignin.configure({
// [Android] what API you want to access on behalf of the user, default is email and profile
webClientId:
'Your---ID.googleusercontent.com', // client ID of type WEB for your server (needed to verify user ID and offline access)
offlineAccess: true, // if you want to access Google API on behalf of the user FROM YOUR SERVER
});
const signIn = async () => {
try {
await GoogleSignin.hasPlayServices();
GoogleSignin.signIn().then(data => {
const currentUser = GoogleSignin.getTokens().then(res => {
console.log('Accesstoken:' + res.accessToken); //<-------get accesstoken="" already="" authenticate="()" available="" cancelled="" catch="" console.log="" const="" e.g.="" else="" error.code="==" error="" et="" flow="" idtoken:="" idtoken="" if="" in="" is="" login="" not="" operation="" or="" outdated="" play="" progress="" res.idtoken="" services="" sign="" statuscodes.in_progress="" statuscodes.play_services_not_available="" statuscodes.sign_in_cancelled="" the="" user=""> {
Backend logic
};
return (
<SafeAreaView>
<StatusBar barStyle="dark-content" backgroundColor="#ece8ff" />
<View style={styles.surface}>
<View style={{flex: 3, backgroundColor: '#ece8ff'}}>
<View
style={{
paddingVertical: 100,
paddingHorizontal: 30,
maxWidth: '90%',
marginTop: 20,
}}>
<Text
style={{
fontSize: 30,
fontWeight: 'bold',
color: '#110c66',
}}>
React native google sign in tutorial
</Text>
<Text style={{paddingTop: 20}}>
Please sign in to continue, its simply very fast and easy and
takes less than a second usually with google sign in.
</Text>
</View>
</View>
<View style={styles.Googlebutton}>
<GoogleSigninButton
style={{width: 240, height: 48}}
size={GoogleSigninButton.Size.Wide}
color={GoogleSigninButton.Color.Dark}
onPress={signIn}
/>
<Text style={{paddingTop: 60, fontWeight: '600'}}>
By signing you agree to our
</Text>
<Text style={{fontWeight: '600'}}>
Privacy Policy and Terms and Conditions
</Text>
<Text style={{paddingTop: 20, fontWeight: '300'}}>
Made with{' '}
<MaterialCommunityIcons name="heart" color={'red'} size={16} /> for
world
</Text>
</View>
</View>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
surface: {
backgroundColor: '#ece8ff',
height: '100%',
},
Googlebutton: {
flex: 3,
justifyContent: 'center',
alignItems: 'center',
marginHorizontal: 15,
backgroundColor: '#fff',
borderRadius: 30,
marginVertical: 60,
},
});
export default inject('store2')(observer(Auth));
-------get>
0 Response to Google Authentication in React native app with access and id token
Comments are personally moderated by our team. Promotions are not encouraged.
Post a Comment